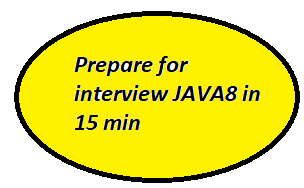
Stream API – All methods with code example. All under one roof .
import Util.Employee;
import java.util.*;
import java.util.function.*;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import java.util.stream.Stream;
import java.util.stream.Stream.Builder;
public class Main {
public static void main(String[] args) {
Employee employee = new Employee();
List<Employee> emplList = employee.getEmpList();
//1. Stream<T> filter(Predicate<? super T> predicate);
//2. Stream<T> distinct(); intermediate operation
//3. void forEach(Consumer<? super T> action); //Terminal operation
//4. void forEachOrdered(Consumer<? super T> action);
List<Integer> integerList = Arrays.asList(1, 5, 9, 2, 3, 2, 3);
List<Integer> evenNumbers = integerList.stream().distinct().filter(i -> i % 2 == 0).collect(Collectors.toList());
System.out.println(“Even Number: “);
System.out.println(evenNumbers);
System.out.println(“Unique value”);
List<Integer> uniqueList = integerList.stream().distinct().collect(Collectors.toList());
uniqueList.forEach(i ->System.out.println(i));
//convert in set , it will be unique list
System.out.println(“Set has only unique value so convert it in set”);
Set<Integer> uniqueSet = integerList.stream().collect(Collectors.toSet());
integerList.stream().forEachOrdered(i ->System.out.println(i)); // forEachOrdered doesnot work on list directly, it works on stream only.
//5. <R> Stream<R> map(Function<? super T, ? extends R> mapper);
List<Integer> squareList = integerList.stream().map(n -> n * n).collect(Collectors.toList());
System.out.println(“Square of each number is :”);
squareList.forEach(i ->System.out.println(i));
List<String> empNameList = emplList.stream().map(e -> e.getName()).collect(Collectors.toList());
System.out.println(“Emp names are:”);
empNameList.forEach(e ->System.out.println(e));
//6. IntStream mapToInt(ToIntFunction<? super T> mapper);
//LongStream mapToLong(ToLongFunction<? super T> mapper);
//DoubleStream mapToDouble(ToDoubleFunction<? super T> mapper);
IntStream empSalaryList = emplList.stream().mapToInt(e -> Math.toIntExact(e.getSalary()));
System.out.println(“Emp salaries are:”);
empSalaryList.forEach(s ->System.out.println(s));
//7. <R> Stream<R> flatMap(Function<? super T, ? extends Stream<? extends R>> mapper);
List<List<Integer>> listOfOrders = Arrays.asList(Arrays.asList(1, 2, 3), Arrays.asList(4, 5, 6), Arrays.asList(7, 8));
List<Integer> allOrderNos = listOfOrders.stream().flatMap(l -> l.stream()).collect(Collectors.toList());
System.out.println(“All ordernos are:”);
allOrderNos.forEach(o ->System.out.println(o));
//8. IntStream flatMapToInt(Function<? super T, ? extends IntStream> mapper);
//LongStream flatMapToLong(Function<? super T, ? extends LongStream> mapper);
//DoubleStream flatMapToDouble(Function<? super T, ? extends DoubleStream> mapper);
System.out.println(“All ordernos are, coverted by flatMapToInt:”);
IntStream allInFlat = listOfOrders.stream().flatMapToInt(l -> l.stream().mapToInt(i -> i.intValue())); //.mpaToInt(Integer::intValue)
//int[] arrayList = allInFlat.toArray(); // you can convert this to array also
// If you uncomment that , that will throw exception : java.lang.IllegalStateException: stream has already been operated upon or closed
allInFlat.forEach(i ->System.out.println(i));
//9. Stream<T> sorted();
System.out.println(“Integer in sorted form :”);
List<Integer> sortedList = integerList.stream().sorted().collect(Collectors.toList());
System.out.println(“Integer List in sorted form :”);
sortedList.forEach(e ->System.out.println(e));
// List<Employee> sortedEmp = emplList.stream().sorted().collect(Collectors.toList());
// System.out.println(“Employee in sorted form :”);
//sortedEmp.forEach(e -> System.out.println(e.toString())); //Exception in thread “main” java.lang.ClassCastException: Util.Employee cannot be cast to java.lang.Comparable
List<Employee> sortedEmp = emplList.stream().sorted(Comparator.comparingInt(a -> a.getEmpId())).collect(Collectors.toList());
System.out.println(“Employee in sorted form :”);
sortedEmp.forEach(e -> System.out.println(e.toString()));
//10. Stream<T> peek(Consumer<? super T> action); // this is used for debugging purpose
System.out.println(“Demo of peek method”);
emplList.stream().peek(e ->System.out.println(e)).collect(Collectors.toList());
//11. Stream<T> limit(long maxSize);
// Stream<T> skip(long n);
System.out.println(“Top 2 records ,using limit method”);
List<Integer> top2Records = integerList.stream().limit(2).collect(Collectors.toList());
top2Records.forEach(t ->System.out.println(t));
System.out.println(“skip first 2 records ,using skip method”);
List<Integer> skipFirst2 = integerList.stream().skip(2).collect(Collectors.toList());
skipFirst2.forEach(s ->System.out.println(s));
//12. Object[] toArray(); returns an array
//<A> A[] toArray(IntFunction<A[]> generator);
System.out.println(“toArray method”);
Object[] toArr = integerList.stream().toArray();
System.out.println(toArr);
Employee[] empArr = emplList.stream().filter(e -> e.getSalary() > 1000).toArray(Employee[]::new);
System.out.println(empArr);
//13. Optional<T> reduce(BinaryOperator<T> accumulator);
// T reduce(T identity, BinaryOperator<T> accumulator);
//<U> U reduce(U identity, BiFunction<U, ? super T, U> accumulator, BinaryOperator<U> combiner);
System.out.println(“7. Minimum/maximum value, without min method, by reduce method”);
//14. Sum, min, max, average, and string concatenation are all special cases of reduction. //reduce returns optional
Integer minimum = integerList.stream().reduce((a, b) -> a < b ? a : b).get();
System.out.println(minimum);
//sum by reduce method
Integer sum = integerList.stream().reduce(0, (a, b) -> a + b);
Integer sum1 = integerList.stream().reduce(0, Integer::sum);
//product by reduce method
BinaryOperator<Integer> accumulator = (a, b) -> a * b;
Integer product = integerList.stream().reduce(1, accumulator);
System.out.println(“Sum is :” + sum + ” Sum1 is :” + sum1 + ” Product is :” + product);
System.out.println(“convert arrays to String by reduce method :”);
String[] strArray = {“Vicky”, “Dhiraj”, “Bhupen”};
Arrays.stream(strArray).reduce((s1, s2) -> s1 + “,” + s2).ifPresent(s ->System.out.println(s));
System.out.println(“convert List to String by reduce method :”);
List<String> strList = Arrays.asList(“Vickykr”, “Dhiraj”, “Bhupi”);
strList.stream().reduce((x, y) -> x + “,” + y).ifPresent(s ->System.out.println(s));
//15. <R> R collect(Supplier<R> supplier, BiConsumer<R, ? super T> accumulator, BiConsumer<R, R> combiner);
Supplier<Map<Integer, String>> supplier = HashMap::new;
BiConsumer<Map<Integer, String>, String> accumulator1 = (map, name) -> map.put(name.length(), name);
BiConsumer<Map<Integer, String>, Map<Integer, String>> combiner = (map1, map2) -> map1.putAll(map2);
Map<Integer, String> result = strList.stream().collect(supplier, accumulator1, combiner);
System.out.println(“Collect in map :”);
System.out.println(result);
//16. <R, A> R collect(Collector<? super T, A, R> collector);
Map<Integer, String> empMap = emplList.stream().collect(Collectors.toMap(e -> e.getEmpId(), e -> e.getName()));
Map<Integer, String> empMap2 = emplList.stream().collect(Collectors.toMap(Employee::getEmpId, Employee::getName));
System.out.println(empMap);
Map<String, List<Employee>> empMap3 = emplList.stream().collect(Collectors.groupingBy(Employee::getCity));
System.out.println(empMap3);
Map<String, Map<Long, List<Employee>>> empMap4 = emplList.stream().collect(Collectors.groupingBy(Employee::getCity,
Collectors.groupingBy(Employee::getSalary)));
System.out.println(empMap4);
//17.Optional<T> min(Comparator<? super T> comparator); //terminal operation
//Optional<T> max(Comparator<? super T> comparator);
//long count(); //terminal operation
System.out.println(“Minimum/ Maximum”);
Comparator<Integer> integerComparator = Integer::compareTo;
Integer min = integerList.stream().min(integerComparator).get();
Integer max = integerList.stream().max(integerComparator).get();
long count = integerList.stream().count();
System.out.println(“total count is :” + count + ” Min is : ” + min + ” Max is :” + max);
//18. boolean anyMatch(Predicate<? super T> predicate);
//boolean allMatch(Predicate<? super T> predicate);
//boolean noneMatch(Predicate<? super T> predicate);
Predicate<Employee> predicate = e -> e.getName().equalsIgnoreCase(“Vicky”);
boolean anyMatchFound = emplList.stream().anyMatch(predicate);
boolean allMatchFound = emplList.stream().allMatch(predicate);
boolean noneMatchFound = emplList.stream().noneMatch(predicate);
System.out.println(“anyMatchFound : ” + anyMatchFound + ” allMatchFound : ” + allMatchFound + ” nonMatchFound : ” + noneMatchFound);
//19. Optional<T> findFirst(); //terminal operation
//Optional<T> findAny();
Optional<Employee> firstEmpFound = emplList.stream().filter(e -> e.getSalary() == 100).findFirst();
Optional<Employee> empFound = emplList.stream().filter(e -> e.getSalary() == 100).findAny();
System.out.println(“First Emp found : ” + firstEmpFound + ” any emp found : ” + empFound);
//20. public static<T> Builder<T> builder() { return new Streams.StreamBuilderImpl<>(); } // building stream
System.out.println(“Build stream: “);
Builder<String> builder = Stream.builder();
builder.add(“Alice”);
builder.add(“Bob”);
builder.add(“Charlie”);
Stream<String> strStream = builder.build();
strStream.forEach(System.out::println);
//21. public static<T> Stream<T> empty() { return StreamSupport.stream(Spliterators.<T>emptySpliterator(), false); } // returns empty sequential stream
Stream<String> emptyStream = Stream.empty();
System.out.println(“IsEmpty Stream : ” + emptyStream.findAny());
//22. public static<T> Stream<T> of(T t) {return StreamSupport.stream(new Streams.StreamBuilderImpl<>(t), false);} //Returns a sequential Stream containing a single element.
//23. public static<T> Stream<T> of(T… values) { return Arrays.stream(values);} //returns a sequential Stream containing the elements of the specified array.
Stream singleStream = Stream.of(“Alice”);
singleStream.forEach(System.out::println);
Stream streamArray = Stream.of(“Alice”, “Bob”);
streamArray.forEach(System.out::println);
//23.Stream.iterate() method in Java’s Stream class generates a sequential stream of elements by iterating a function over an initial element.
Integer seed = 1;
UnaryOperator<Integer> increment = s -> (s + 1) * 2;
System.out.println(“Stream iterate method”);
Stream.iterate(seed, increment).limit(10).forEach(System.out::println);
//24. Stream.generate () // returns infinite sequential unordered stream
System.out.println(“Stream generate method, generating infinite stream”);
Supplier<Double> randomNumbers = () ->Math.random();
Stream<Double> randomNosStream = Stream.generate(randomNumbers);
randomNosStream.limit(5).forEach(System.out::println);
//25. Stream.concat()
System.out.println(“Stream Concat”);
Stream<String> str1Stream = Stream.of(“Alice”, “Bob”);
Stream<String> str2Stream = Stream.of(“Vicky”, “Rajesh”);
Stream<String> concatStream = Stream.concat(str1Stream, str2Stream);
concatStream.forEach(System.out::println);
//26. public interface Builder<T> extends Consumer<T> // this is part of builder pattern , which is used to create complex object step by step .
//Find Duplicate value
System.out.println(“Find Duplicate value”);
Map<Integer, Long> duplicateMap = integerList.stream().collect(Collectors.groupingBy(n -> n, Collectors.counting()));
duplicateMap.entrySet().stream()
.filter(e -> e.getValue() > 1).forEach(e -> System.out.println(e.getKey()));
}
}